Create Simple HTTP/2 Server With KoaJs
It's been a year (give or take) I've using Express and I just realized that Express still using HTTP/1.x.
Photo by Alternate Skate on Unsplash
Then How to Create HTTP/2 Server on NodeJs?
Once I know that Express still using HTTP/1.x, I start searching on google which framework that use HTTP/2 protocol on NodeJs. Then I found KoaJs. Withouth further a do, I read the documentation and come up with this simple Koa app.
const Koa = require("koa")const app = new Koa()const port = process.env.PORT || 80
app.use(async ctx => { ctx.body = "Hello World!\n"})
app.listen(port, () => console.log("listening on port %i", port))
But when I run the server and make a request, it still use HTTP/1.x
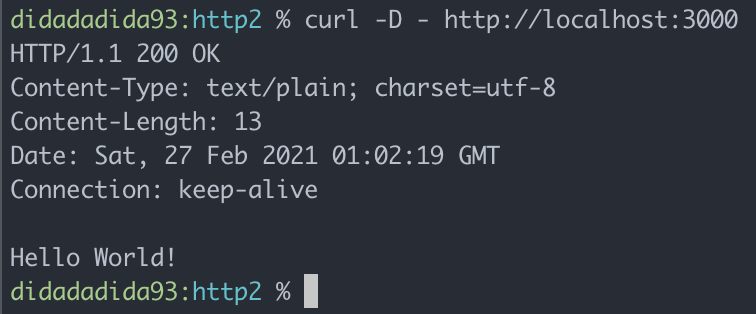
I found that app.listen
is a syntatic sugar for running nodejs http module, so the code will be something like this
const http = require("http")const Koa = require("koa")const app = new Koa()const port = process.env.PORT || 80
app.use(async ctx => { ctx.body = "Hello World!\n"})
http .createServer(app.callback()) .listen(port, () => console.log("listening on port %i", port))
Alright, so I know I can't use app.listen
to start a server. So if I want to run http/2 server, then I need to modify the script a little bit so it start http/2 server.
const fs = require("fs")const http2 = require("http2")const Koa = require("koa")const app = new Koa()const port = process.env.PORT || 80
app.use(async ctx => { ctx.body = "Hello World!\n"})
const options = { key: fs.readFileSync("./private.key"), cert: fs.readFileSync("./cert.crt")}
http2 .createSecureServer(options, app.callback()) .listen(port, () => console.log("listening on port %i", port))
Please note that http2 module required you to run on HTTPS protocol so you need SSL certificate. There is a lot of article that help you to generate self-signed SSL certificate for development purpose.
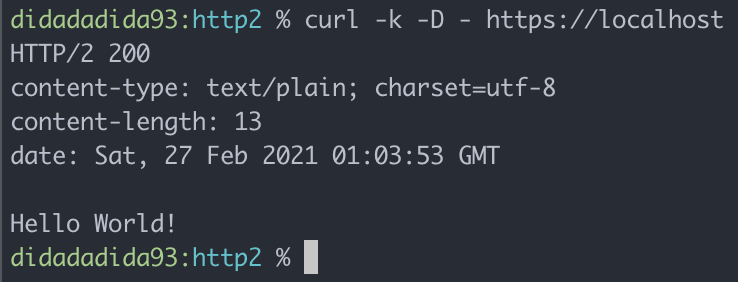
Let's Create Simple Rest API
By default, KoaJs didn't have method for define HTTP verb like ExpressJs. So if I want to create simple rest API using KoaJs but want to feel it like I'm using ExpressJs, I have to install middleware to add this functionality.
In here you will find a lot of middleware that can be use for KoaJs application. As for now I will use @koa/router since this module is provided by KoaJs. I update the code so it look like this
const fs = require("fs")const http2 = require("http2")const Koa = require("koa")const Router = require("@koa/router")const port = process.env.PORT || 443
const app = new Koa()const router = new Router()
router.get("/", (ctx, next) => { ctx.body = { status: "Running!" }})router.get("/songs", (ctx, next) => { ctx.body = JSON.parse(fs.readFileSync("./songs.json"))})
app.use(router.routes())
const options = { key: fs.readFileSync("./private.key"), cert: fs.readFileSync("./cert.crt")}
http2 .createSecureServer(options, app.callback()) .listen(port, () => console.log("listening on port %i", port))
And here is the response from the route that I just made.
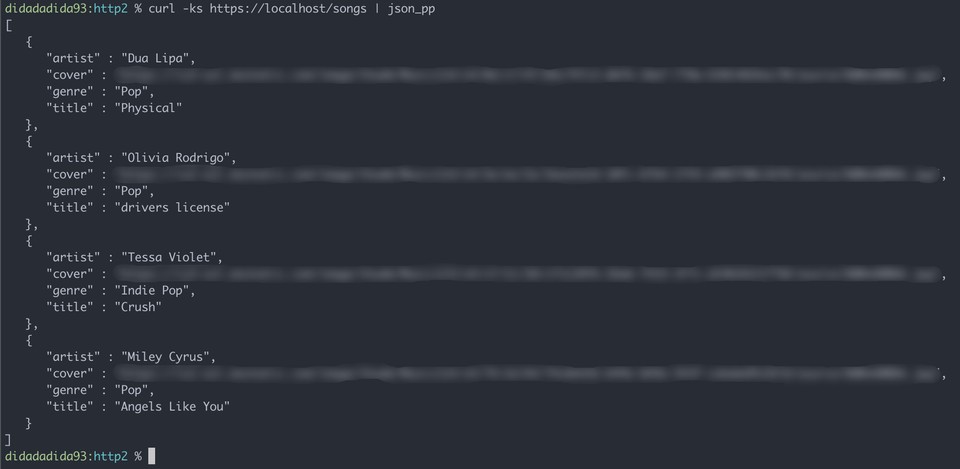
Now we can add new routes just like we using ExpressJs. Yeay 🤩